6 Common Causes of Application Performance Issues
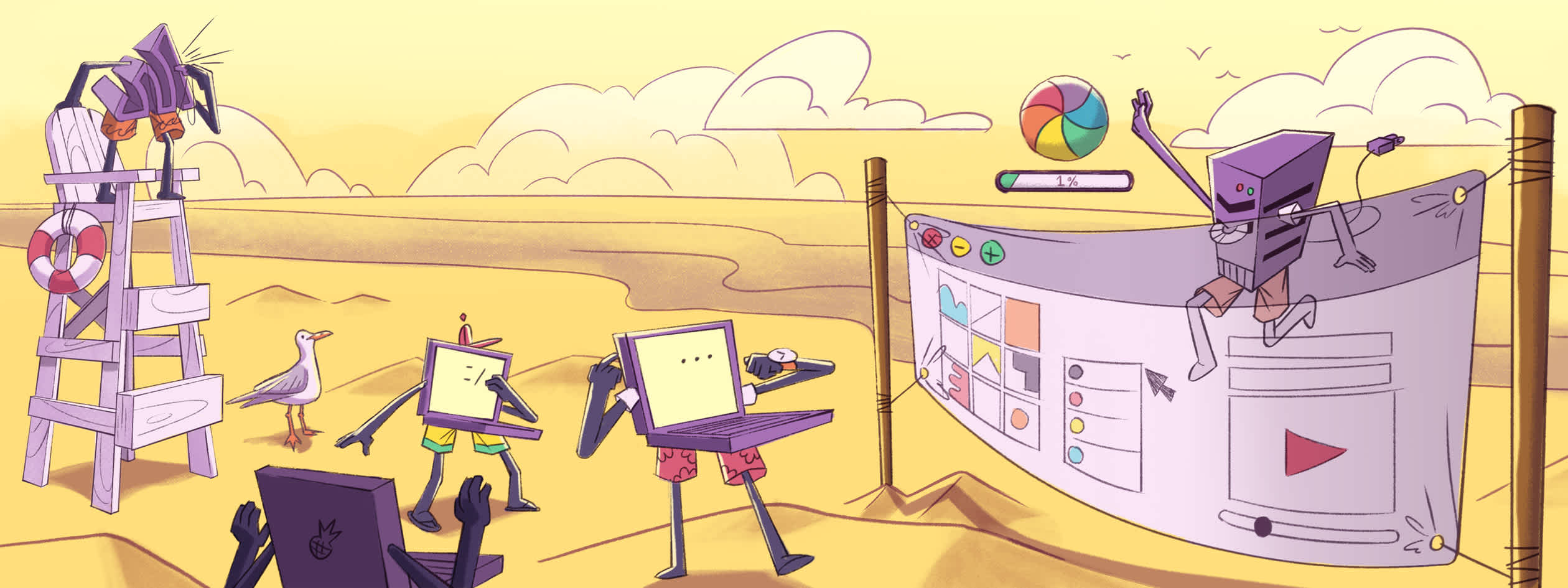
ON THIS PAGE
- 1. Network Latency
- 2. Slow Servers
- 3. Database Issues
- 4. Code Quality
- 5. Misconfiguration
- 6. Third-Party Code Dependencies
- Application Performance and the User Experience
Application performance is critical for a seamless user experience, but all too often, developers find themselves struggling to pinpoint slowdowns. Understanding the root cause is the first step to diagnosing and solving performance issues. In this article, we’ll explore six common root causes of application performance slowdowns and share actionable advice on how to fix them. We will also show how platforms like Sentry can help uncover, trace, and debug these issues faster.
Network latency can significantly degrade application performance, especially in microservices or distributed systems where numerous services communicate with each other. Every request that traverses a network introduces some delay, and these delays can add up quickly if not optimized.
Microservices-based applications, real-time communication apps, and distributed systems that require frequent inter-service data transfers are particularly susceptible to network latency issues. For more information on how to debug microservices architectures, check out this guide.
As an application developer, you do not have control over the network latency of your users, but you can design your application to proactively reduce the impacts of varying latency.
Optimize API Requests: Minimize the payload of requests and implement caching to reduce unnecessary data transfers. Read more about how Dan Mindru was able to cut out 22.3 seconds off an API request in this blog post.
Asynchronous Communication: Where possible, use non-blocking requests for improved parallel processing. For example, avoid render-blocking assets to ensure a quick page load, as suggested by Lazar in this article.
Monitoring and Tracing: Use tools like Error Monitoring to identify latency issues in distributed services and pinpoint where bottlenecks occur. Traces across services provide insight into how long each request takes and where optimizations can be made.
While there are many ways to account for high network latency, persisting connections with a session object reduces the overhead of repeatedly establishing a new connection, improving network performance. For example, in Python:
import requests
# Using a session object to persist connections
with requests.Session() as session:
response = session.get("https://example.com/api/resource")
if response.status_code == 200:
data = response.json()
In his blog, “What’s the difference between API Latency and API Response Time?” Lazar Nikolov discusses latency versus response time and how each impacts overall performance.
Underpowered servers or inefficiently configured environments can lead to slow response times and can impact the performance of the entire application stack. Web applications with high traffic, like e-commerce platforms, and any application that handles intensive backend processing are prone to issues caused by slow servers.
One of the biggest challenges with application slowdowns on the backend is they can be difficult to identify, especially when the slowdown perpetuates to the frontend.
Capacity Planning & Autoscaling: Regularly analyze traffic patterns and configure autoscaling to handle spikes.
Server Configuration: Tune server settings like connection limits, thread pool sizes, and enable Gzip compression to improve response times.
Real-Time Monitoring: Use Sentry’s real-time, full-stack monitoring to track server-side issues and monitor performance trends. This enables a proactive approach to resolving server slowness before it becomes a critical issue.
Distributed Tracing: Use Sentry Tracing to trace slowdowns throughout your entire stack and determine exactly where you should focus on making improvements.
If you’ve identified that it’s your server that is causing a slowdown, you might want to focus improvements on response compression and concurrency. In this NGINX configuration, enabling Gzip compression reduces the size of responses sent to clients, and adjusting worker_connections
can help handle more concurrent connections efficiently.
# NGINX server configuration for optimal performance
worker_processes auto;
worker_connections 1024;
gzip on;
gzip_types text/plain application/json;
Whether it’s your server or a service you’re using that is causing a slowdown, learn how to trace the issue to the root cause with Salma’s post on improving Time To First Byte.
Poorly optimized queries, inadequate indexing, and database misconfigurations can significantly slow down your application, leading to slow page loads and delayed API responses. Applications with complex queries, high write/read operations, or a large user base, such as content management systems, social media platforms, and SaaS applications, are highly impacted by database performance issues.
Once you have identified that the slowdown is happening with your database, there are many things you can do to make improvements, here are a few common issues that you will likely want to check:
Query Optimization: Use indexes, analyze execution plans, and avoid N+1 query patterns.
Caching Data: Use tools like Redis or Memcached for frequently accessed data.
Performance Tracing: Leverage Sentry’s Query Performance Tracking to identify slow database queries and their impact on application performance. By tracing queries in context, you can easily determine which parts of your database schema or application code are causing bottlenecks.
Optimizing database queries will be very dependent on your data and how your application uses your data. A rule of thumb is to make sure you’re requesting what data you need as specifically as possible. For example, adding an index to the email column improves SQL query performance by reducing the time required to locate rows in large tables.
-- Optimize query with an index
CREATE INDEX idx_user_email ON users(email);
SELECT * FROM users WHERE email = 'example@example.com';
Poorly written code often leads to inefficiencies, such as excessive memory usage, unnecessary loops, and inefficient data structures, which can slow down the entire application. Applications with large codebases or those that require frequent releases and updates, such as web applications and enterprise software, often suffer from performance issues related to poor code quality.
Code quality is often about the practice across your engineering team, but some tools can still help you identify opportunities for improvements.
Code Coverage: Ensuring you have code coverage (tests) across your system is critical for catching code quality issues. Codecov has a helpful piece on “Defining High Quality Code.”
Code Profiling: Identify and optimize slow parts of your code using a performance profiler. With Sentry Profiling you can quickly see your most regressed functions and get to the line of code causing a slowdown.
Refactor Regularly: Maintain clean code practices and perform regular refactoring to enhance performance. Learn how Clean Architecture makes debugging easier in this recent post from Lazar.
Error Monitoring & Alerts: Use Sentry’s Insights to track and manage performance-related errors in your codebase. Sentry’s context-rich insights help you understand not just where errors happen but also their root cause.
While identifying larger issues such as waterfalls can be made easy with debuggability tools like Sentry, there are also opportunities to always improve code as you refactor and grow your code base. For example, by handling promises in JavaScript in parallel versus in series, you can improve the overall performance of your application. Here’s an example in Python:
// Iterating over each item causes promises to be handled in series
const items = await getItems()
for (let item of items) {
await processItem(item)
}
// Using Promise.all allows promises to be handled in parallel
const items = await getItems()
await Promise.all(items.map(processItem))
Incorrect configurations can lead to inefficient resource use, security vulnerabilities, and reduced performance. This can include everything from misconfigured database connections to inappropriate server settings. Web servers, cloud-hosted applications, and microservices architectures that rely heavily on environment variables and configuration files are most at risk.
Regardless of whether an issue is coming from your code, the services you’re using, or the way you have configured your deployments, it is critical you have a system that is easily debuggable and reliably monitored. Here are a few ways to ensure dependable uptime.
Automated Configuration Management: Use tools like Ansible or Terraform to enforce configuration standards.
Test Changes Safely: Implement configuration changes in a staging environment before rolling out to production.
Identify Misconfigurations Quickly: Sentry’s Release Tracking and Uptime Monitoring enables you to detect and resolve configuration issues as soon as they are deployed, reducing the risk of misconfiguration-related performance issues.
Something that can get missed is deployment configurations. For example, by setting appropriate memory and CPU limits for a Kubernetes pod, you can prevent resource contention and optimize application performance.
# Kubernetes Deployment with optimized resource limits
resources:
limits:
memory: "512Mi"
cpu: "500m"
requests:
memory: "256Mi"
cpu: "250m"
Dependencies can introduce unexpected performance issues, particularly if they are outdated, not maintained, or perform blocking operations that slow down your application. Apps that rely on multiple third-party libraries, integrations, or external APIs, such as SaaS applications, content aggregators, and web platforms, are particularly susceptible to this root cause.
While nearly every application relies on third-party dependencies, they do create additional challenges with regard to performance. External services are beyond your control, and these upstream dependencies can produce application performance issues that are hard to identify, and even harder to fix. Here are some tips for capturing and resolving third-party dependency issues quickly.
Regular Dependency Audits: Regularly update and audit third-party libraries to prevent performance and security issues.
Isolate Third-Party Calls: Use background workers or async processing to prevent blocking operations.
Leverage Spans: Sentry Tracing supports custom spans for monitoring Third-Party Services, allowing you to trace how third-party code impacts your app and quickly identify problematic dependencies.
One way to ensure your main thread isn’t blocked by third-party dependencies is to use asynchronous processing for API calls. Here’s an example using JavaScript:
// Use asynchronous call to prevent blocking
async function fetchData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
processData(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
Today's users are more demanding and unforgiving than ever, so the secret to a positive digital experience is to catch and resolve performance problems before the user notices.
How do you achieve this ambitious goal? This blog's catalog of potential root causes of application performance issues makes it clear: delivering an exceptional user experience requires a diligent, proactive, multi-faceted approach that includes full-stack monitoring, alerting, root cause analysis, and debugging.
Using a comprehensive debuggability platform like Sentry enables developers to discover, trace, and resolve performance issues efficiently. By taking a holistic approach to network latency, server configuration, database performance, code quality, misconfiguration, and third-party dependencies, you can ensure that your application remains fast, efficient, and reliable.
Learn more about improving your application’s performance with these resources:
Also, feel free to reach out to us on Discord, GitHub, or X if you’re ready to get started with performance monitoring.