Debugging a Django Application
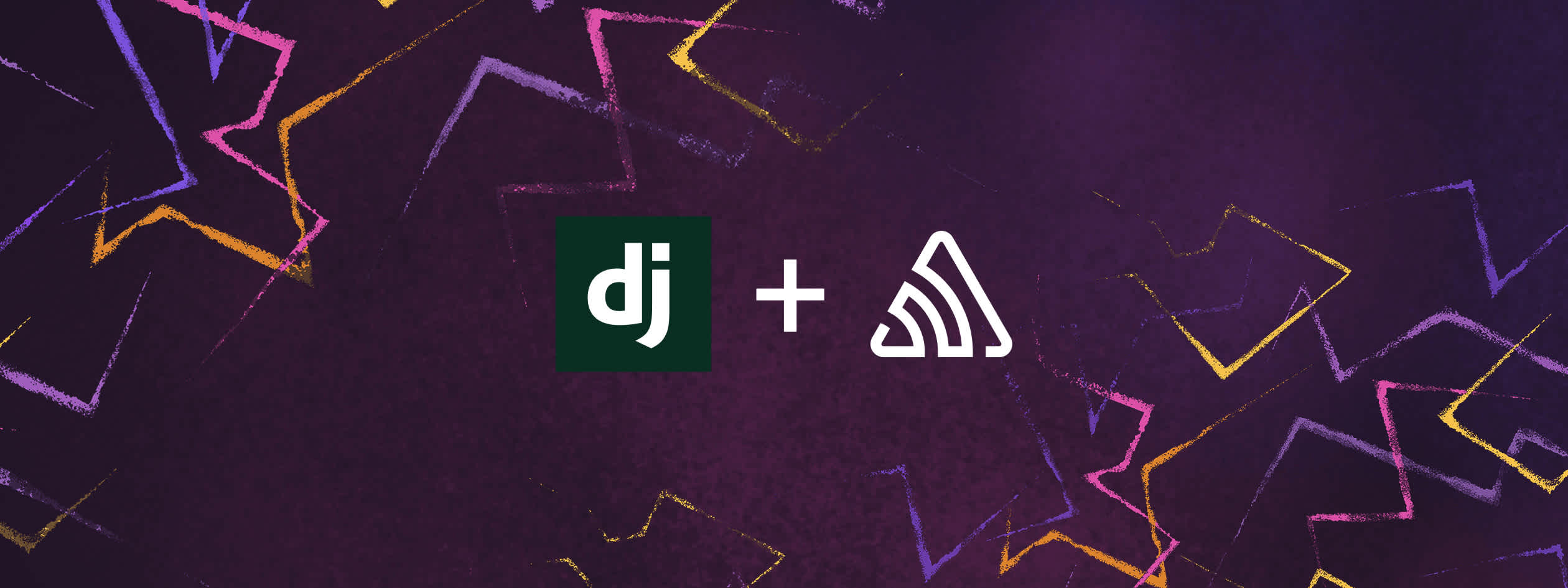
Debugging Django applications can be challenging, but it’s key to keeping your app running smoothly in production. From unexpected bugs to performance slowdowns, finding and fixing issues efficiently keeps users happy and reduces downtime. For example, when an error occurs on a critical page, like a checkout page, identifying the issue quickly is crucial to avoid disrupting user transactions. In this article, we’ll look at how to use Sentry for error monitoring in Django, giving you real-time insights into application health and error reporting. Whether it’s minor bugs or critical failures, these tips will help you speed up debugging in Django so you can quickly identify the root cause of issues and prioritize fixes to keep your Django app performing at its best.
You may be researching something as simple as “What is the best way to debug a Django application?” Or you’re curious to learn about any Django-specific tools to help you debug in Django. Then again, maybe you’re looking to answer super-specific questions like, “Can you debug a template that is taking too long to render? Or why is an API endpoint crashing? Is there a better way to debug than using print
statements?”
Regardless of what brought you here, there are a bunch of tools available to help debug a Django application. Here are a few of the more popular ones:
Django Shell
Django shell is a Python shell that lets us access the database API included with Django. When we open the Django shell, Django loads all dependencies for the project and imports Django settings, allowing us to evaluate expressions related to our project.
We can start the Django shell using the manage.py
file, like so:
$ python manage.py shell
Django Debug Toolbar
The Django Debug Toolbar is a visual tool that helps us debug the Django application.
The Django debug toolbar offers information on every page of our app using a sliding sidebar. It gives us information about various parts of the app, like the current request/response, resource usage (e.g. time), Django settings, HTTP headers, SQL queries, cache, logging, and more.
We can install the toolbar with pip
:
(venv) $ pip install django-debug-toolbar
Now, we need to add it to the project’s INSTALLED_APPS
:
# settings.py INSTALLED_APPS = [ 'my_project', ... 'debug_toolbar' ]
Then, we will need to add it to the application’s MIDDLEWARE_CLASSES
:
# settings.py MIDDLEWARE_CLASSES = [ 'django.contrib.auth.middleware.SessionAuthenticationMiddleware', ... 'debug_toolbar.middleware.DebugToolbarMiddleware' ]
The toolbar’s sidebar will appear on any connection that matches Django’s INTERNAL_IPS
if the DEBUG
value is set to True
.
Django PDB
Python’s built-in debugging module pdb
is an excellent tool to debug any Python application interactively.
Django PDB is a package that allows us to use the pdb
module in the context of Django applications. It automatically activates the pdb
for any endpoint.
We can install the django-pdb
module using pip
:
(venv) $ pip install django-pdb
Next, we need to add it to the end of the application’s INSTALLED_APPS
:
# settings.py INSTALLED_APPS = [ 'my_project', ... 'django_pdb' ]
Then, we will need to add it to the end of the application’s MIDDLEWARE_CLASSES:
# settings.py MIDDLEWARE_CLASSES = [ 'django.contrib.auth.middleware.SessionAuthenticationMiddleware', ... 'django_pdb.middleware.PdbMiddleware' ]
Django PDB is fairly easy to use if you are familiar with Python’s pdb
module. You can learn more about its usage in the documentation
.
IDE Debug Tools
Lastly, we can also use the built-in debugging features of the IDE or code editor to debug a Django application.
Here are some links to debug a Django app in different IDEs and code editors:
Final Thoughts on Debugging Django Apps
Debugging Django applications doesn’t have to be a daunting task. With the right tools and techniques, developers can streamline the process, quickly identify the root causes of issues, and deploy fixes with confidence. Sentry offers powerful features for tracking errors, monitoring performance, and gaining visibility into application health, making it a valuable resource for maintaining Django applications. By integrating Sentry into your debugging workflow, you not only reduce troubleshooting time but also improve the overall stability and performance of your application. With these practices in place, you’re well-equipped to deliver a seamless, reliable experience to your users and keep your Django application running at its best.
If you’re ready to learn some new techniques to debug your Django applications, be sure to give Sentry a try. Our blog post entitled “Django Performance Improvements - Part 1: Database Optimizations” is a great place to start. And be sure to visit our docs to learn even more. If you have any feedback, please feel free to join the discussion on Discord or GitHub.