Practical Tips on Handling Errors and Exceptions in Python

Abdul D -
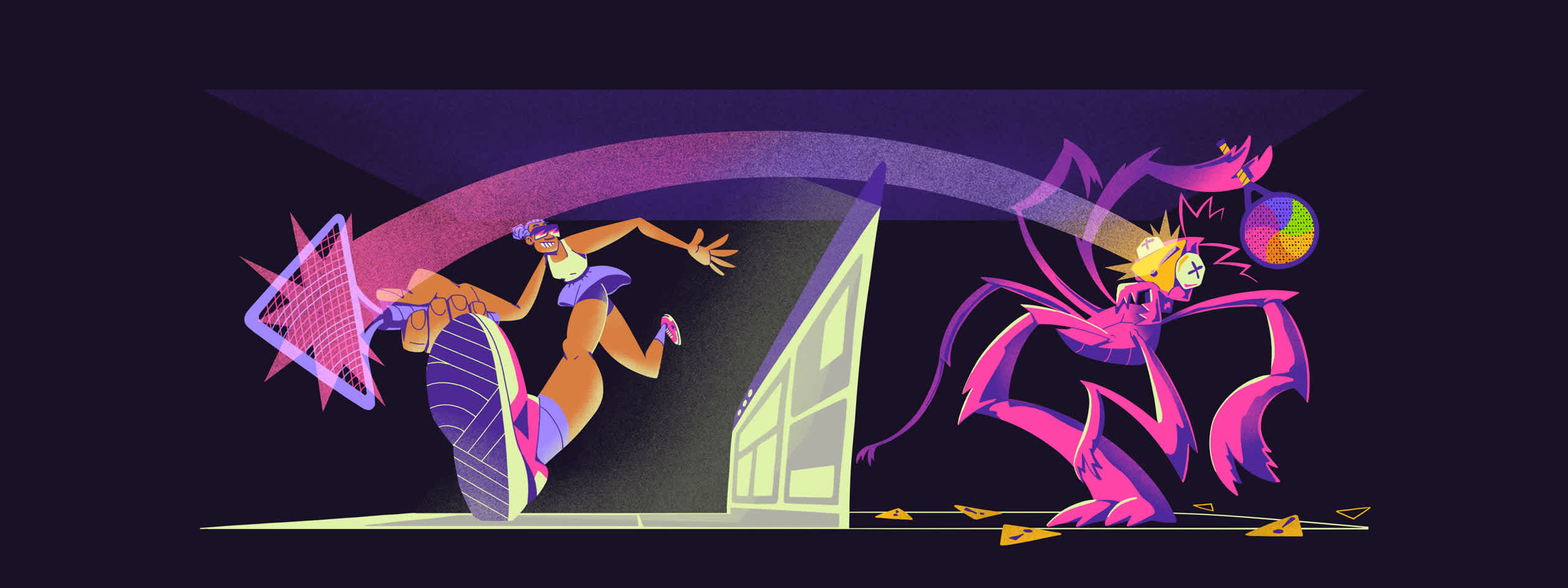
ON THIS PAGE
- Errors vs Exceptions in Python: What’s the Difference?
- Errors in Python
- Exceptions in Python
- Why Is Exception and Error Handling Important in Python?
- How Sentry Can Help With Error Monitoring in Your Python Applications
Have you ever encountered a confusing error message that left you wondering what went wrong in your Python code? You’re not alone. Even the most experienced developers run into exceptions, making it essential to understand how to handle them effectively.
While basic syntax errors can be caught early by code editors and debugging tools, more complex issues often arise at runtime, requiring a structured approach to exception handling.
Regardless of experience level, every Python developer can benefit from mastering exception handling. This guide will help you understand the difference between errors and exceptions, explore common types of exceptions, and learn best practices for handling them in your Python applications.
We’ll also cover how Sentry can help you monitor and track exceptions in real time, providing detailed insights into your application’s performance and stability.
Sentry tracing
Writing flawless code is an unrealistic expectation, and every developer will inevitably encounter unpredictable behavior in their programs due to coding mistakes. These issues typically fall into two categories: errors and exceptions.
Errors are fundamental coding mistakes that prevent a program from running altogether. Errors are commonly detected during compilation, and the code won’t execute until they are fixed.
Exceptions are a subcategory of errors and occur during program execution (runtime) when your code encounters an unexpected situation, such as trying to divide by zero.
result = 10 / 0
# Raises ZeroDivisionError
Unlike errors, exceptions can be caught and handled within your code.
Error handling and debugging are closely related but serve different purposes in the development process.
Error handling is proactive in that you anticipate potential issues in code and implement mechanisms to prevent crashes and provide meaningful feedback to users.
Debugging, on the other hand, is reactive: It involves identifying and resolving issues after they occur. Debugging tools like breakpoints, logging, and stack traces help you understand what went wrong and how to fix it.
Errors like syntax errors occur when the Python interpreter detects an issue during parsing, before execution even begins, preventing the program from running altogether. Because these errors reflect fundamental problems in the code structure, they must be fixed before execution can proceed.
Syntax and indentation errors are among the most common in Python and prevent the code from being interpreted into bytecode.
A SyntaxError occurs when Python cannot parse code because it doesn’t follow the correct syntax rules. For example, say you try to run a Python script containing the following line of code that is missing a closing parenthesis:
print("Hello World" # Missing closing parenthesis
The Python interpreter would return the following error message:
File "example.py", line 2
SyntaxError: unexpected EOF while parsing
An IndentationError occurs when Python expects an indented block of code, but the indentation is either missing or incorrect. For example, the code below throws an IndentationError: expected an indented block because of the incorrectly indented print statement.
def greet(): print("Hello")
Syntax and indentation errors often occur when writing new code or making changes to existing code. They can be frustrating, but they’re also easy to fix once you understand what the error message is telling you.
An exception is a type of error that occurs during program execution, disrupting the normal flow of code. When an exception is raised, Python halts execution and creates an exception object containing details about what went wrong. The interpreter then searches for an appropriate except block to handle the exception. If no such block is found, Python terminates execution and provides a traceback message to help debug the issue.
Python provides some built-in exception classes to handle different error scenarios. Let’s take a look at some of the most common exceptions you’ll encounter.
TypeError:
Raised when an operation is performed on incompatible types, such as trying to add a string and an integer.Click to Copyprint("10" + 5) # Raises TypeError
ValueError:
Occurs when a function receives an argument with a value not suitable for the operation being performed, such as trying to cast a string of non-numeric characters to an integer.Click to Copynum = int("abc") # Raises ValueError
KeyError
: Raised when trying to access a non-existent dictionary key.Click to Copydata = {"name": "Alice"} print(data["age"]) # Raises KeyError
IndexError
: Occurs when accessing an out-of-range index in a list or array.Click to Copynumbers = [1, 2, 3] print(numbers[5]) # Raises IndexError
ZeroDivisionError:
Triggered when attempting to divide by zero,Click to Copyresult = 10 / 0 # Raises ZeroDivisionError
OverflowError:
Triggered when the resulting value exceeds the allowed number range.Click to Copyimport math print(math.exp(1000)) # Raises OverflowError
FileNotFoundError:
Raised when attempting to access a non-existent file.Click to Copywith open("missing_file.txt", "r") as file: content = file.read() # Raises FileNotFoundError
You can define custom exception classes to extend Python’s Exception class and handle specific cases in your application.
class CustomError(Exception): pass raise CustomError("This is a custom exception!")
When your Python code runs into an exception, understanding what went wrong is key to fixing the problem. Exception details provide information about the type of error, where it occurred in your code, and why it happened.
When Python encounters an exception at runtime, it generates an exception object containing:
The type of exception, for example, ZeroDivisionError.
A description of what went wrong, for example, division by zero.
The traceback, which shows exactly where the exception occurred in the code.
Traceback (most recent call last): File "example.py", line 1, in <module> print(5 / 0) ^^^^^^^^^^^^ ZeroDivisionError: division by zero
You can extract information from the exception object and print it as an error message by catching the exception details in an except _____ as block, for example:
try: print("10" + 5) except Exception as e: print(f"Exception Type: {type(e).__name__}") # Gets the type of exception print(f"Exception Message: {e}") # Gets the error message
This code prints a nicely formatted error message:
Exception Type: TypeError Exception Message: can only concatenate str (not "int") to str
The Python traceback module provides a standard interface for extracting, formatting, and printing detailed information about exceptions and errors, including the call stacks. This provides an in-depth view of what went wrong, including the exact line number where the error occurred. Tracebacks are especially useful when debugging complex issues or when you need to understand the context of an error. With a view of the call stack, you can pinpoint the root cause of an issue. The example code below shows how to use the traceback module to log a traceback when an exception occurs:
import traceback try: num = int("abc") except ValueError as e: print("An error occurred!") traceback.print_exc() # Prints full traceback information
Python’s exception handlers allow you to gracefully handle issues and provide alternative execution paths instead of abruptly terminating the program. Exception handlers are especially useful for dealing with unexpected errors in user input, file operations, or network requests, allowing the program to continue running instead of crashing.
The try block allows you to execute code that may raise an exception, while the except block catches and handles any exceptions that occur. In addition to ensuring the program continues running when an exception is raised, this structure provides a way to view the details of the exception.
try: data = {"name": "Max Verstappen"} print(data["age"]) # This will raise KeyError because "age" is not in the dict except KeyError as e: print(f"Error: {e}")
You can extend the try block with else and finally blocks to provide extra functionality. An else block executes code only if no exception occurs, while a finally block runs regardless of whether an exception is raised.
For example, in the following code snippet, a file is opened in a try block. If no exception occurs, the else block reads the file’s contents and closes it.
try: file = open("example.txt", "r") except FileNotFoundError: print("File not found!") else: print("File opened successfully!") content = file.read() print(content) file.close() finally: print("End of file operation.")
You can catch multiple exception types by chaining except blocks or using a tuple in an except block.
For example, to handle both ValueError and ZeroDivisionError, you can do the following:
try: x = int(input("Enter a number: ")) print(10 / x) except (ValueError, ZeroDivisionError) as e: print(f"Error: {e}")
Errors displayed in the console are cleared when the program terminates. If you want to keep a record of your program’s execution for later analysis, you can use the logging module to log errors to a file instead of printing them to the console.
import logging logging.basicConfig(filename='errors.log', level=logging.ERROR) try: result = 10 / 0 except ZeroDivisionError as e: logging.error(f"Error occurred: {e}")
Here, errors are logged to an errors.log file that you can analyze to understand what went wrong. The Python logging module is powerful and can be configured to log errors at different levels and send them to various destinations. You can learn more about logging and debugging in our Python Logging Guide.
You can define custom exception classes to extend Python’s Exception class and handle specific cases in your application. These custom, application-specific exception classes allow you to tailor exception handling to your application use case, make error messages more meaningful, and simplify debugging.
class InvalidAgeError(Exception): pass age = -1 if age < 0: raise InvalidAgeError("Age cannot be negative!")
Tools like Pylint and flake8 can help detect syntax and indentation issues before execution. These work similarly to a spell checker for your code, helping you catch errors early before they cause runtime issues.
Effective error and exception handling can determine whether an application is robust or crashes frequently. Here’s why implementing proper error handling is essential.
When an unhandled exception occurs, Python terminates the program immediately. By handling an exception, you can prevent the program from crashing and provide a graceful recovery mechanism.
while True: try: value = int(input("Enter a number: ")) print(f"100 divided by {value} is equal to: {100 / value}") break except ZeroDivisionError: print("Cannot divide by zero!") except ValueError: print("Invalid input! Please enter a number.")
In this example, the program prompts the user to enter a number. If the user inputs 0, the program catches the ZeroDivisionError and displays a friendly message instead of crashing. If the user enters a non-numeric value, the program catches the ValueError and prompts the user to enter a valid number.
In both cases, the program continues running without crashing until the value variable is valid, and then it breaks out of the loop.
Python Exception Handling
By handling exceptions, the program remains stable and returns meaningful information, which can help you understand what went wrong and rectify the issue.
Displaying informative error messages can help your users understand what went wrong and how they can correct their input or actions. For example, if a user tries to open a non-existent file, you can catch the FileNotFoundError exception and display a message indicating that the file does not exist.
try: with open("nonexistent_file.txt", "r") as file: content = file.read() except FileNotFoundError: print("Error: The file you are trying to open does not exist.")
Instead of showing a cryptic traceback, the user gets a clear message about the missing file.
Exception handling allows you to log errors for later analysis, helping to pinpoint the source of an issue. Logging is especially useful for debugging applications running in production, where you can’t interactively debug the code. For more information on logging in Python, check out our Python Logging Guide.
Handling exceptions properly ensures that resources like open files, database connections, and network sockets are properly closed, preventing memory leaks and data corruption. While memory management is automatic in Python, resource cleanup is not, so it’s essential to close resources explicitly. You can ensure proper resource cleanup by using with blocks or context managers.
For example, if your project uses an API key stored as an environment variable, you might want to avoid the situation where that key gets logged when an API call fails.
Consider the following:
# assume the following variable is set in your environment
# export SECRET_API_KEY=this-is-a-secret
import os import requests api_key = os.getenv("SECRET_API_KEY") r = requests.get(f"https://api.example.com/customer/1234?API_KEY={api_key}")
If the HTTP request fails,, you’ll see an error that contains something like this in your logs:
raise ConnectionError(e, request=request) requests.exceptions.ConnectionError: HTTPSConnectionPool(host='api.example.com', port=443): Max retries exceeded with url: /customer/1234?API_KEY=this-is-a-secret (Caused by NameResolutionError("<urllib3.connection.HTTPSConnection object at 0x103d2ecf0>: Failed to resolve 'api.example.com' ([Errno 8] nodename nor servname provided, or not known)"))
Note that we can see the key this-is-a-secret in the error log, which is what we want to avoid.
By handling the exception properly, we can avoid this. A simple example is as follows:
__# assume this variable is set in your environment __# export SECRET_API_KEY=this-is-a-secret import os import requests api_key = os.getenv("SECRET_API_KEY") try: r = requests.get(f"https://api.example.com/customer/1234?API_KEY={api_key}") except requests.exceptions.ConnectionError: print("Connection error when accessing api.example.com")
Now we’ll only see the following output if the request fails:
Connection error when accessing api.example.com
This is less informative but more secure. In a real-world case, you’d need to think carefully about what information you want logged when things go wrong to balance making debugging easy while avoiding cases of leaking any sensitive information.
In a production environment, it can be difficult to gain clear insights into how your application is performing, especially when errors or issues arise. Without proper visibility, diagnosing and fixing problems becomes a time-consuming and frustrating process, which could lead to increased downtime, unhappy users, or missed opportunities for improvement.
Sentry addresses these challenges by enhancing the monitoring of Python applications. It provides a comprehensive, real-time view of exceptions, errors, and performance issues within your production environment. Rather than just reporting vague error messages or logs, Sentry captures detailed contextual information, including stack traces, and even the specific conditions under which an error occurred. This rich set of data helps developers understand not only what went wrong but also the context in which the problem occurred, making it easier to reproduce, diagnose, and fix issues quickly.
Let’s simulate an exception and capture it using Sentry. First, you need to install the Sentry SDK for Python:
pip install sentry-sdk
You’ll need a Sentry account and project to get a DSN (Data Source Name) key. Find detailed instructions on how to set up Sentry for Python in the Sentry for Python documentation.
Now we’ll create a ZeroDivisionError for Sentry to capture:
import sentry_sdk sentry_sdk.init("your-dsn") try: result = 10 / 0 except ZeroDivisionError as e: sentry_sdk.capture_exception(e) print("Exception captured and sent to Sentry.")
Run this code and Sentry will capture the exception. You can view the issue in your Sentry dashboard.
Sentry exception tracking
Click on an issue to view further information about the exception, including the stack trace:
Zero division error details
Sentry provides an extensive breakdown of each issue, including frequency, affected users, and stack traces. Using Trace View, developers gain deeper insights into how an exception propagates through an application. The breadcrumb feature provides a detailed timeline of events leading up to an error, helping to pinpoint root causes more effectively.
The example below uses a transaction to track more information about the event. A transaction represents a single instance of an activity you want to measure or track, like a page load, page navigation, or an asynchronous task. Transaction information helps you monitor the overall performance of your application beyond when it crashes or generates an error. Without transactions, you can only know when things in your application have actually gone wrong, which is important, but not the whole picture.
import sentry_sdk from sentry_sdk import start_transaction sentry_sdk.init( dsn="<your-dsn>", send_default_pii=True, # Set traces_sample_rate to 1.0 to capture 100% # of transactions for tracing, but make sure to # set it to much lower (ex. 0.15) for production # so you don’t quickly fill up your events quota.. traces_sample_rate=1.0, ) def create_new_user(new_user): # Simulate an exception, e.g. user already exists if new_user["id"] == 123: raise Exception("User already exists") else : return new_user if __name__ == "__main__": with start_transaction(name="user_signup_process"): try: data = { "id": 123, "email": "demo@example.com" } user = create_new_user(data) except Exception as e: print(f"An error occurred: {e}") sentry_sdk.capture_exception(e)
(note for in-production environments, you should set it to something lower, like 0.15, so you don't use up all your events quota.)
Running this code will produce an exception:
An error occurred: User already exists
And Sentry will capture this exception and provide more insights into the issue:
User signup exception trace
The Trace View provides a detailed breakdown of where and when errors occur within your stack trace.
General trace view
To learn more about Trace View and how tracing can help you understand the context of errors in your application, check out the Trace View documentation.
Beyond tracking errors, Sentry allows you to monitor application performance in real time. By capturing performance metrics, you can identify the impact of errors on your application’s performance and user experience.
Performance metrics
Sentry collects an aggregated view of errors and exceptions, allowing you to track issues by number of events, affected users, and more. This helps you prioritize issues based on their impact on the user experience.
Issues dashboard
Sentry also features a customizable dashboard that displays key metrics and performance data, helping teams identify trends and prioritize issues effectively.
Dashboard chart
Sentry simplifies debugging and improves the developer workflow, making it an invaluable tool for teams focused on building stable, high-performance Python applications. Sentry even has python ai autofix functionality to fix your code before it affects your users.
To explore more, check out our Python documentation or sign up to get started.